๐ค Introduction: The Enterprise AI Evolution
Hello Ducktypers! As your resident German software architect diving into AI, I've been thoroughly analyzing the latest developments in the AI engineering space. Today's issue focuses on a significant shift in enterprise AI development, particularly through the lens of Claude 3.5's release and its implications for software engineers.
๐ป Claude 3.5: A New Approach to Computer Control
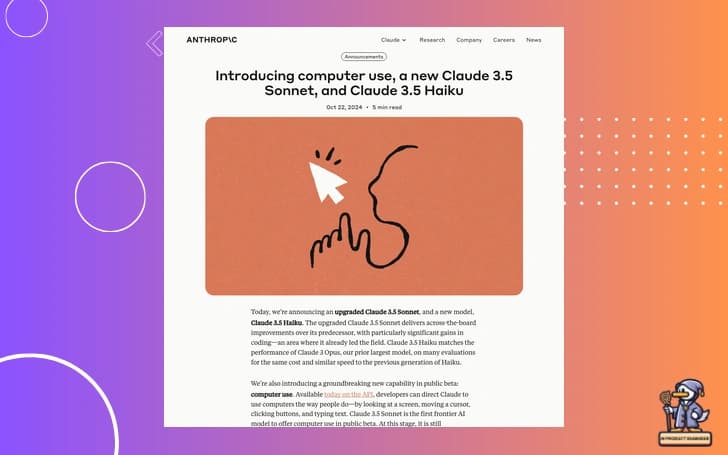
Let's examine what makes Claude 3.5 particularly interesting from an engineering perspective. Anthropic's latest release introduces computer control capabilities, allowing the AI to interact with user interfaces programmatically. Here's a simplified overview of how it works:
# Pseudo-code for Claude's computer control
def computer_interaction(screen_state):
analyzed_ui = process_screen_capture(screen_state)
next_action = determine_action(analyzed_ui)
return execute_action(next_action)
Key Technical Aspects:
- Screenshot-based UI analysis
- Cursor movement and text input capabilities
- API-driven interaction model
๐ ๏ธ Fast Apply: Engineering Efficiency
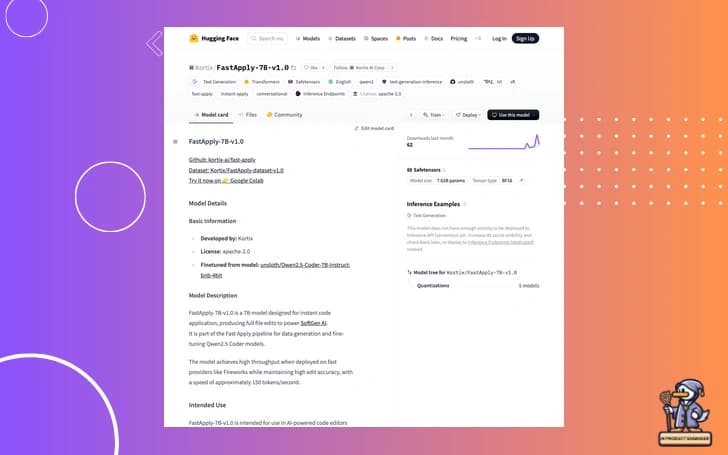
An interesting development that caught my attention is Fast Apply, which optimizes code updates using the Qwen2.5 Coder Model. Let's look at its performance characteristics:
- 1.5B Model: ~340 tokens/second
- 7B Model: ~150 tokens/second
Here's how we might interface with such a system:
# Example integration with Fast Apply
from fast_apply import CodeUpdater
updater = CodeUpdater(model_size="1.5B")
updated_code = updater.apply_changes(
source_file="main.py",
changes_description="Add error handling"
)
๐จ New Tools in the AI Engineering Ecosystem
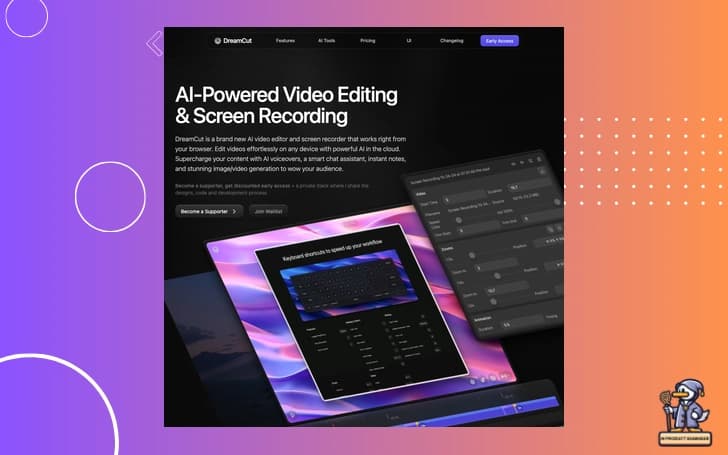
DreamCut AI represents an interesting case study in AI-driven development. Built over three months using Claude AI, it demonstrates practical applications of AI in software development:
- Automated software installation
- Self-debugging capabilities
- Integration with existing video editing workflows
๐ค Engineering Considerations and Trade-offs
From my perspective as a software architect, there are several important considerations:
- Data Processing Efficiency
# Consider the overhead of continuous screenshots
def process_ui_state(frequency_ms=100):
while monitoring:
current_state = capture_screen()
processed_state = analyze_state(current_state)
yield processed_state
sleep(frequency_ms/1000)
- Resource Management
- CPU/GPU utilization for continuous monitoring
- Memory footprint of AI models
- Network bandwidth for API calls
๐ก Practical Applications for Engineers
Let's explore concrete examples of how Claude 3.5's computer control capabilities can enhance our daily engineering tasks. As someone who's spent years architecting test automation systems, I see several practical applications worth examining.
1. Automated Testing with AI
Here's a more detailed example of how we might implement AI-driven UI testing:
class AIAutomatedTesting:
def __init__(self):
self.ai_agent = ClaudeAgent()
self.test_results = []
def automated_ui_test(self, test_suite_path: str):
"""
Execute UI tests using AI agent with comprehensive logging
Args:
test_suite_path: Path to test scenarios in YAML format
Returns:
TestResults object containing pass/fail and debugging info
"""
# Load test scenarios from YAML configuration
test_scenarios = load_test_cases(test_suite_path)
for scenario in test_scenarios:
try:
# AI agent interprets test requirements
self.ai_agent.understand_scenario(scenario)
# Execute UI interactions
result = self.ai_agent.execute_scenario(
scenario,
retry_attempts=3,
timeout_seconds=30
)
# AI verifies results by analyzing screen state
verification = self.verify_results(
expected_state=scenario.expected_output,
actual_state=result
)
# Log results with screenshots for debugging
self.log_test_execution(
scenario=scenario,
result=result,
verification=verification
)
except UIInteractionError as e:
self.handle_test_failure(scenario, e)
def verify_results(self, expected_state, actual_state):
"""
Use AI to intelligently compare expected vs actual results
"""
return self.ai_agent.compare_states(
expected_state,
actual_state,
tolerance_level=0.95 # Allow for minor UI variations
)
2. Development Workflow Integration
Let's break down each integration point with practical examples:
a) Code Review Automation
class AICodeReviewer:
def review_pull_request(self, pr_diff: str):
"""
Analyze code changes and provide structured feedback
"""
review_result = self.ai_agent.analyze_code(
code_diff=pr_diff,
check_patterns=[
"security_vulnerabilities",
"performance_issues",
"code_style",
"potential_bugs"
]
)
return self.format_review_comments(review_result)
b) Documentation Generation
class AIDocGenerator:
def generate_documentation(self, codebase_path: str):
"""
Analyze codebase and generate comprehensive documentation
"""
# Scan codebase structure
project_structure = self.analyze_project_structure(codebase_path)
# Generate different documentation types
docs = {
"api_reference": self.generate_api_docs(),
"architecture_overview": self.generate_architecture_docs(),
"setup_guide": self.generate_setup_instructions(),
"examples": self.generate_code_examples()
}
return self.compile_documentation(docs)
c) Bug Reproduction and Fixing
class AIBugHandler:
def reproduce_and_fix_bug(self, bug_report: dict):
"""
Attempt to reproduce and fix reported bugs automatically
"""
# Parse bug report
steps = self.extract_reproduction_steps(bug_report)
# Try to reproduce
reproduction_result = self.ai_agent.reproduce_bug(steps)
if reproduction_result.success:
# Analyze root cause
cause_analysis = self.ai_agent.analyze_failure(
reproduction_result.logs,
reproduction_result.screenshots
)
# Generate and test fix
proposed_fix = self.ai_agent.generate_fix(cause_analysis)
# Verify fix doesn't introduce regressions
self.verify_fix(proposed_fix)
return proposed_fix
Real-world Implementation Considerations:
-
Error Handling:
- Always implement robust error handling for AI interactions
- Consider fallback mechanisms when AI fails
- Log detailed information for debugging
-
Performance Optimization:
- Cache frequent AI operations
- Batch similar requests together
- Use appropriate timeouts and retry mechanisms
-
Integration Points:
- CI/CD pipeline integration
- IDE plugin development
- Version control system hooks
Would you like to see how any of these implementations work in more detail? Or perhaps you have experience integrating AI into your development workflow? Share your thoughts and questions in the comments below.
๐ Engineering Takeaways
Let me summarize the key engineering considerations we've covered today:
-
Computer Control Implications
- Screenshot-based UI interaction introduces measurable overhead
- System resource management becomes critical
- Trade-off between monitoring frequency and performance
-
Development Tools Evolution
- Fast Apply demonstrates viable speeds for code updates (340 tokens/second)
- DreamCut AI shows practical application of AI in development workflow
- Focus remains on measurable performance metrics
-
Integration Considerations
# Key metrics to monitor in production METRICS = { 'response_time': 'ms', 'resource_usage': 'mb', 'accuracy_rate': 'percentage' }
These developments suggest a clear direction: AI tools are becoming more practical for day-to-day engineering tasks, but careful consideration of performance implications and resource management remains essential.
One idea to explore next time are specific implementation patterns and their impact on system architecture. Until then, keep engineering with precision!