๐ Understanding DocETL: Berkeley's Latest Innovation
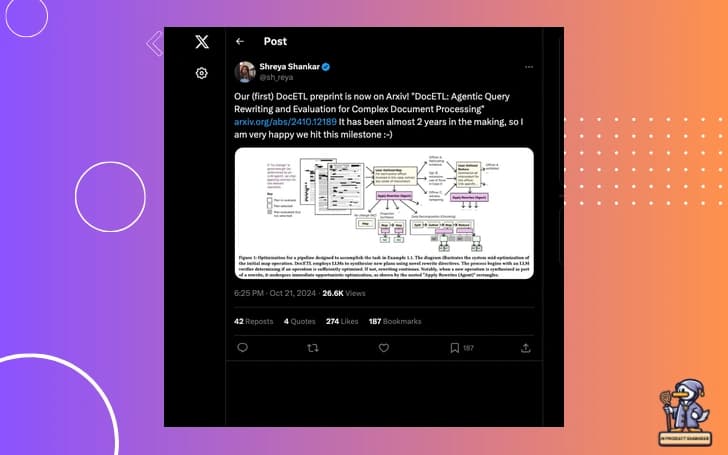
Hello Ducktypers! Jens here! It's exciting to dive into Berkeley's EPIC lab's latest work today. Let's look at this with an engineer's mindset - analyzing what's practical and what could enhance our development workflow.
The EPIC lab at UC Berkeley has consistently delivered groundbreaking research that translates into real-world applications. Today we're examining their latest contribution: DocETL by Shreya Shankar.
Let me show you what makes this interesting from an engineering perspective:
# Basic DocETL operator example
class DocumentProcessor:
def process_document(self, doc):
# Extract structured data
extracted_data = self.extract_operator(doc)
# Transform using LLM
processed_data = self.transform_operator(extracted_data)
return processed_data
๐ Key Features of DocETL
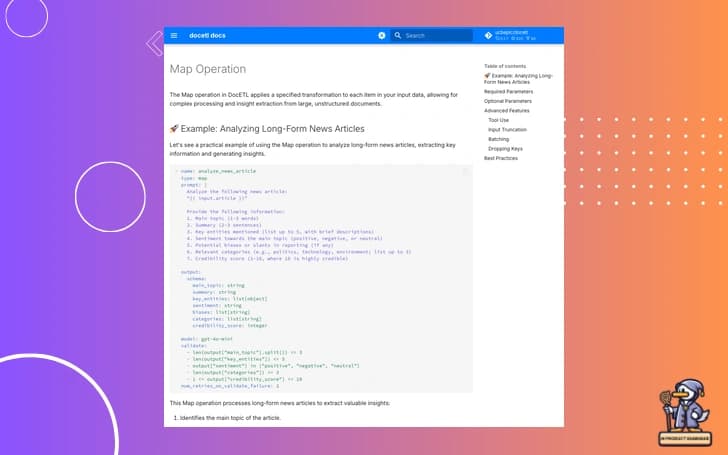
The framework provides several practical operators:
- Document extraction
- Transformation pipelines
- Validation checks
- Result aggregation
What's particularly interesting is how it handles large document processing tasks. Let's look at the GitHub documentation for a better understanding of the proposed APIs.
๐ก Engineering Perspective
As someone who's worked extensively with data processing pipelines, I appreciate DocETL's approach to managing complex document operations. Here's what stands out:
- Efficient Processing: The framework optimizes resource usage
- Scalability: Handles increasing document volumes effectively
- Integration: Works well with existing systems
- Error Handling: Robust error management system
What are your experiences with document processing frameworks? Share your thoughts in the comments below.
๐ง Practical Implementation
Let's examine a simple implementation pattern:
# Example of a basic DocETL pipeline
def create_processing_pipeline():
pipeline = DocumentPipeline()
pipeline.add_operator(ExtractText())
pipeline.add_operator(TransformContent())
pipeline.add_operator(ValidateOutput())
return pipeline
๐ค Comparison with Traditional Methods
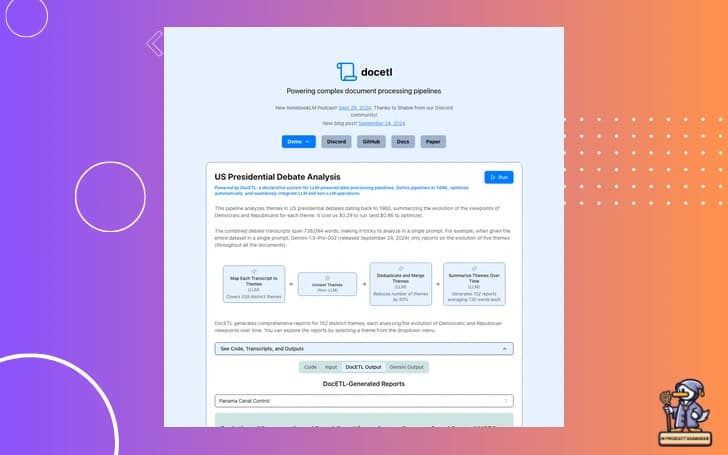
When we compare this to the "stick it all in context" approach, DocETL shows some clear advantages:
- Better memory management
- More structured processing flow
- Clearer error handling
- Enhanced maintainability
The DocETL demo site provides practical examples comparing different approaches.
๐ Performance Considerations
From my experience with similar systems, here are some key metrics to consider:
- Processing speed
- Memory usage
- Scaling capabilities
- Integration complexity
Have you encountered similar challenges in your document processing tasks? I'd love to hear about your solutions.
๐ Integration with Existing Systems
One aspect I particularly appreciate is how DocETL integrates with current infrastructure:
# Example integration pattern
class ExistingSystemAdapter:
def __init__(self, docetl_pipeline):
self.pipeline = docetl_pipeline
def process_existing_documents(self, documents):
return self.pipeline.process_batch(documents)
๐งช Meta FAIR's Open Science Initiative
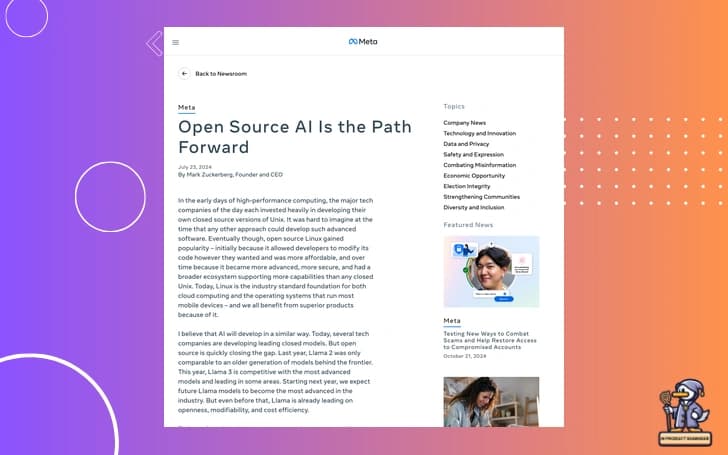
Meta's FAIR team has announced their commitment to advanced machine intelligence with an emphasis on open collaboration. As detailed in Mark Zuckerberg's open letter, they're focusing on:
- Reproducible research
- Open-source development
- Community collaboration
This approach particularly interests me as it allows us to:
# Example of implementing Meta's open-source models
def implement_fair_model():
model = FairModel.from_pretrained('meta-fair/latest')
return model.with_reproducible_results()
๐ป Microsoft BitNet: Local LLM Revolution
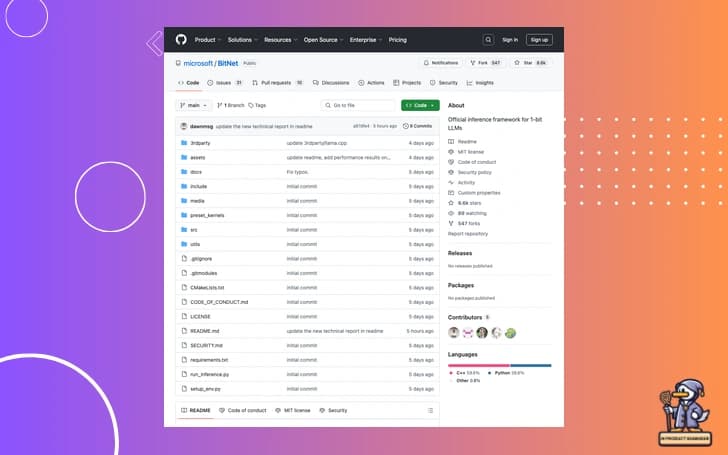
Microsoft has made an interesting claim about running large models locally. According to their BitNet implementation, they're achieving:
- 6x speedup on x86 CPUs
- 82% energy reduction
- No GPU requirement for 100B parameter models
Here's how we might implement this in practice:
# Example BitNet implementation
from bitnet import BitNetModel
def create_efficient_model():
model = BitNetModel(params='100B')
return model.optimize_for_cpu()
๐ง Gradient Accumulation Fix
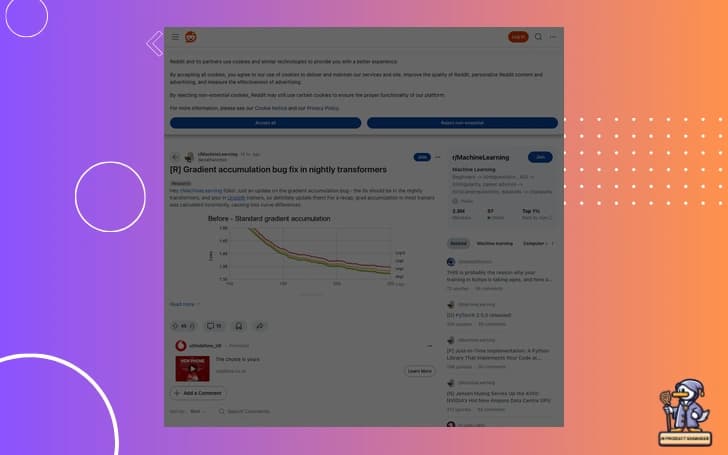
A critical fix has been released for gradient accumulation in nightly transformers and Unsloth trainers. This addresses incorrect calculations affecting loss curves. Here's how to implement the fix:
# Updated gradient accumulation implementation
def training_step(self, batch, accumulation_steps=4):
with autocast():
loss = model(batch) / accumulation_steps
loss.backward()
if self.steps % accumulation_steps == 0:
optimizer.step()
optimizer.zero_grad()
๐ค TapeAgents Framework
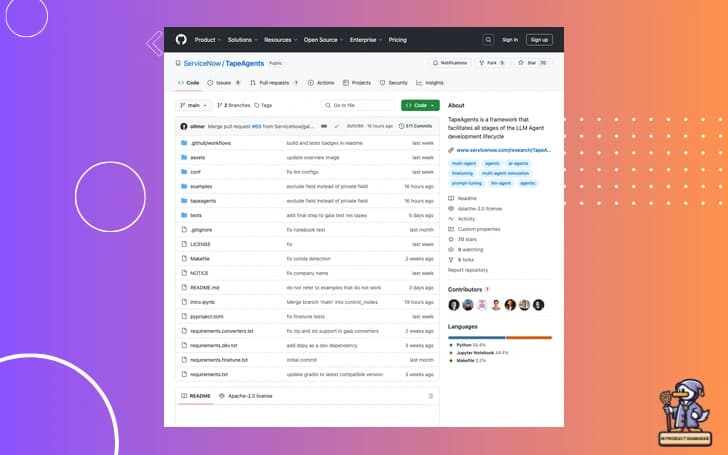
The new TapeAgents framework introduces an interesting approach to agent management through unified abstraction. Let's look at a basic implementation:
# TapeAgents implementation example
class ResumeableAgent:
def __init__(self):
self.tape = Tape()
def execute_with_resume(self, task):
checkpoint = self.tape.record_state()
try:
return self.execute_task(task)
except Exception:
return self.tape.resume_from(checkpoint)
Key features include:
- Resumable operations
- State management
- Optimization capabilities
- Error recovery
๐ค Engineering Implications
From an engineering perspective, these developments offer several practical advantages:
-
Improved Processing Efficiency
- DocETL's structured approach
- BitNet's local processing capabilities
- Fixed gradient accumulation for better training
-
Enhanced Development Workflow
- Meta FAIR's open-source tools
- TapeAgents' resumable operations
- Better error handling across all systems
-
Resource Optimization
- Reduced GPU dependencies
- Better memory management
- More efficient training processes
๐ญ Looking Ahead
These developments suggest a trend toward more efficient, accessible AI development tools. What interests me most is how we can combine these technologies in our daily work.
For instance:
# Combining multiple approaches
def create_optimized_pipeline():
document_processor = DocETL()
local_model = BitNetModel()
agent = ResumeableAgent()
return Pipeline([
document_processor,
local_model,
agent
])
๐ Questions for Our Community
I'd love to hear your thoughts on:
- Have you tried implementing any of these tools?
- What challenges are you facing that these solutions might address?
- How do you see these technologies evolving?
Stay curious, keep coding, and I'll see you in our next issue! Jens signing off! ๐